Billing Address Same as Shipping Address
If you want to set the Billing Address Same as Shipping Address, then you are at the Right Place.
Because in this Tutorial we have created an HTML Webpage to answer your Question.
Here using this CODE, we can copy or Fill the Any Input Field the Same as Shipping Address with a single button click.
index.html : Billing Address Same as Shipping Address
<!DOCTYPE html>
<html>
<body>
<textarea id="ShippingAd"></textarea>
<br/>
<input type="checkbox" id="myCheck" onclick="myFunction()">
<label for="myCheck">Same as Shipping Address:</label>
<br/>
<textarea id="BillingAd"></textarea><br/>
<script>
function myFunction() {
var checkBox = document.getElementById("myCheck");
var textShip = document.getElementById("ShippingAd");
var textBil = document.getElementById("BillingAd");
if (checkBox.checked == true){
textBil.value=textShip.value;
} else {
textBil.value="";
}
}
</script>
</body>
</html>
Output
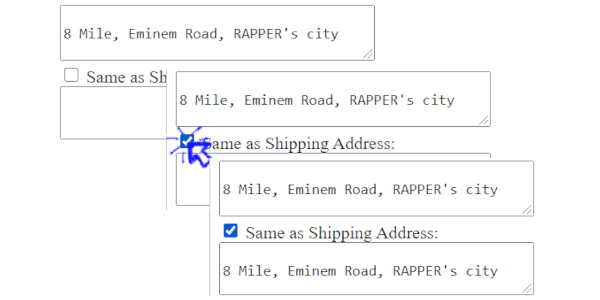
JavaScript Explained :
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
myFunction()
Using Input box’s onClick event, we will call this function [onclick="myFunction()"]
Which includes Some Variables and If Else Condition.
Variables [ var ] for Input, Shipping and Billing Inputs
By using this var checkBox we will store our Input box Element [Check Box Input] by using this CODE.
index.js
var checkBox = document.getElementById("myCheck");
Where document.getElementById(“myCheck”) will grab the HTML element where Element ID is myCheck.
And Same for
index.js
var textShip = document.getElementById("ShippingAd");
var textBil = document.getElementById("BillingAd");
IF ELSE Condition
Using IF Else condition we will check waters CheckBox is checked or NOT.
For that we are giving here this Condition
if (checkBox.checked == true){
}
else {
}
Where checkBox.checked will return TRUE or FALSE on Checking/Unchecking the Input Check Box
Copying Input Values to Billing Address textBil.value=textShip.value;
Using this link textBil.value=textShip.value;
We will Put Shipping Address Value (textShip.value) inside Billing Address (textBil.value) Text Field.
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
textBil.value="";
And by using this line textBil.value="";
We are clearing or Putting Empty String inside our Billing Address Text Feild whenever user uncheck the Input Box.
Whole Code – [Line By Line]
First we have 2 Text Area with ID ShippingAd and BillingAd.
index.html
<textarea id="ShippingAd"></textarea>
<textarea id="BillingAd"></textarea>
And 1 Input checkBox with myCheck ID and onClick Attribute/Event.
index.html
<input type="checkbox" id="myCheck" onclick="myFunction()">
Where using this onClick we are calling our JavaScript Function.
And at our JavaScript Function we have 3 JavaScript Variable.
index.html
var checkBox = document.getElementById("myCheck");
var textShip = document.getElementById("ShippingAd");
var textBil = document.getElementById("BillingAd");
Where Variable checkBox will store Input Checkbox Element,
Variable textShip will store Shipping Address text Field
AND
Variable textBil will store Billing Address text Field.
After that we have IF ELSE condition
Where our Condition is
index.js
if (checkBox.checked == true){
textBil.value=textShip.value;
}
Where this will check that our Input Check box Checked or Not (checkBox.checked == true)
And if it returns TRUE (Means User cliked/Checked the Input Box)
It will copy the Shipping Address Text Field Data into Billing Address text Feild using this line.
index.js
textBil.value=textShip.value;
And if its got Unchecked by User then it will run this Else Condition.
index.js
else {
textBil.value="";
}
Where it will clear Billing Input text Field data using this Line.
index.js
textBil.value="";
Where "" means Empty String. It will replace Billing Text Field Data with Empty String.
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.