How to Play Audio in HTML using JavaScript | Audio Player
In some Case we Don’t want to Show Default Controls that provided by our Browsers to show HTML audio tag’s Data and Wish to Control HTML Audio Data using Custom Controls.
So if you are wonder about How to Play Audio in HTML using JavaScript, Then This Tutorial is for you.
Where we will discuss about everything that you need to know while working with HTML Audio Elements in JavaScript.
Methods to Play Audio in HTML using JavaScript
There are several Methods to Play Audio in HTML Using JavaScript. Where we can call a JavaScript function to play our Audio Data in our HTML Document.
Which can be Done by JavaScript Click Event or HTML’s onClick Attribute.
Method 1 : Playing Audio in HTML using JavaScript and HTML onClick Attribute
To Play HTML Audio using JavaScript and HTML onCLick Attribute we need to create a Function, Which will run using HTML onClick attribute.
As we all know that using HTML’s onClick attribute we can Run JavaScript function.
But first we need to add Some JavaScript Code inside that Function to Play Audio File.
We have to use JavaScript document.getElementById() and .play() Method.
Where document.getElementById() will select the Audio Tag’s Data (Our Audio File) and .play() Method will Play the Selected Elements Data (Audio in this Case).
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play Audio</button>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
Method 2 : Playing Audio in HTML using JavaScript Click Event
If you don’t like to use HTML onClick attribute to run an JavaScript Function, Then you can use JavaScript’s Event Listener to run a specific JavaScript task on a Button Click.
JavaScript’s addEventListener will monitor any Click on the Selected HTML Element.
If someone clicks the Selected HTML Element (Like Button). This will Play the HTML Audio.
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<button id="myBtn">Play Audio</button>
<script> document.getElementById("myBtn").addEventListener("click",function(){
document.getElementById("myAudio").play();
}
);
</script>
</body>
</html>
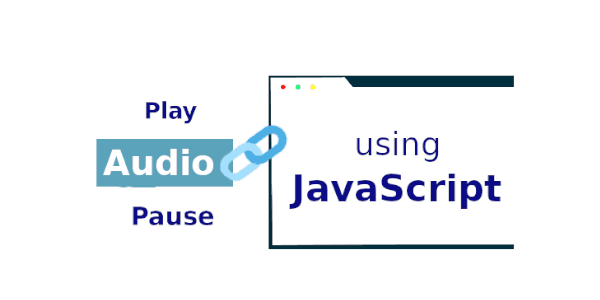
Play and Pause Audio in HTML Using JS
In our last 2 Examples, we are able to play our Audio file.
But there isn’t anything that can stop that audio from playing.
So this Example will cover the Full process to Play and Pause Audio in HTML.
{Note : To make this Example Short we are using onClick Attribute/Event}
First we will add our Audio file inside our HTML Document using HTML’s Audio Tags.
<audio></audio>
After that we will assign our Audio file’s Path using HTML Audio tag’s SRC Attribute.
index.html [Audio Tag Syntax]
<audio src="audioPath"></audio>
Now we will create two HTML Button and add onClick Event to it, So JavaScript can Run the Given Function on a Button Click.
index.html [Assigning Click Events]
<button onClick="function1()">Play</button>
<button onClick="function2()">Pause</button>
index.js
function function1(){
play();
}
function function2(){
pause();
}
index.html [Play and Pause Audio in HTML using JS]
<html>
<body>
<audio src="https://programminghead.com/audio/audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play Audio</button>
<button onClick="pauseMyAudio()">Pause Audio</button>
<p id="audioStatus">Click The Play Button</p>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
document.getElementById("audioStatus").innerHTML="Audio is Playing";
}
function pauseMyAudio(){
document.getElementById("myAudio").pause();
document.getElementById("audioStatus").innerHTML="Audio Paused";
}
</script>
</body>
</html>
FAQ - How to play audio in HTML using JavaScript
How to play and pause audio in JavaScript
To play and pause the audio in HTML using JavaScript, we have .play() and .pause() functions.
As you can tell that by using .play() function we can play our Audio file and by using .pause() function we can pause the audio file.
But before using it, you need to select the audio element and then you can use it with that Audio element.
[Here we are going to use HTML Audio Element where the id for our audio Element is myAudio. This id we will use later to select this audio element inside JavaScript. We are using two Button (play and pause) to call our JavaScript functions by using JS onClick Event and inside our Function we are selecting the Audio Element and by using .play() and .pause() functions we are playing and pausing the audio].
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<button onClick="playAudio()">Play</button>
<button onClick="pauseAudio()">Pause</button>
<script>
function playAudio(){
document.getElementById("myAudio").play();
}
function pauseAudio(){
document.getElementById("myAudio").pause();
}
</script>
</body>
</html>
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
How to play audio in JavaScript
To play audio in JavaScript, we need to call the .play() function in JavaScript.
Just select your Audio Element by using document.getElementById("elementID") and then play the audio using JavaScript .play() Function.
[Impotent: You can't run .play() function automatically, you you need to run use JavaScript ClickEvent to call a function and inside that function you can use .play function to play the audio).
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play Audio</button>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
.play() JavaScript
.play() JavaScript is used for playing the Audio. Where we need to select the HTML Audio Element first, then we can use this .play() JavaScript function to play the Audio.
So just select HTML Audio element by using ID (document.getElementById("audioTagID")) then use this .play() to play the audio. That's it.
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play Audio</button>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
How to pause audio in JavaScript
To pause audio in HTML by using JavaScript, we can use .pause() function with our Audio Element.
So in JavaScript we need to select the Audio element first, the we can pause the audio by using .pause() function.
index.html
<audio src="audio.mp3" id="myAudio"></audio>
<button onClick="pauseAudio()">Play Audio</button>
<script>
function pauseAudio(){
document.getElementById("myAudio").pause();
}
</script>
JavaScript play sound onclick
To play sound onclick in javaScript, we need to use onClick event handler. There are different ways to use onClick Event Handler in JavaScript.
Using onClick inside HTML Element:
In HTML, we can also use JavaScript onClick event inside HTML Element's starting tag. By using onClick event inside HTML Element we can call any JavaScript function on a button click.
IN this case, we will play a sound on button click by using JavaScript onClick Event inside HTML Button.
index.html
<html>
<body>
<audio src="https://programminghead.com/audio/audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play Audio</button>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
Using JavaScript addEventListener to add Click event to HTML Button
If you want to use onClick Event inside HTML Elements, then you can also add an Event Listener to any HTML Element by using .addEventListener().
And after that you need to specify the Event ("click") and then it will listen for future clicks and on click it will execute the given Statements (In our case its .play() for playing the audio sound).
index.html
<html>
<body>
<audio src="https://programminghead.com/audio/audio.mp3" id="myAudio"></audio>
<button id="myBtn">Play Audio</button>
<script>
document.getElementById("myBtn").addEventListener("click", function(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
HTML play sound on button click
If you want to play a sound on a button click in HTML, then you can use JavaScript onClick event. Where by using this onClick event inside HTML element will fire the given function on a Button click.
SO by using onCLick event inside HTML we can call a JavaScript function and through that function we can play the Audio file.
index.html
<html>
<body>
<audio src="https://programminghead.com/audio/audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play Audio</button>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
HTML play sound onclick image
To play sound when you click the HTML image, then you can simply use JavaScript onClick event in your Image and then by using that Image, you can play the Audio file.
First we will add the onClick Event to our IMG tag, then we will call and JavaScript, inside our JavaScript function we will select the Audio element and play the song using .play() function.
index.html
<html>
<body>
<audio src="https://programminghead.com/audio/audio.mp3" id="myAudio"></audio>
<img src="https://www.programminghead.com/logo/logo.png" onClick="playMyAudio()"/>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
Call JavaScript function from HTML
To call a JavaScript function from HTML, we need to use onClick inside HTML Element's Starting Tag.
Then inside onClick we need to write our JavaScript function name and you are DONE.
index.html
<button onClick="myFunction()">RUN</button>
<script>
function myFunction(){
alert("You just RAN this Function using HTML Button");
}
</script>
Which HTML attribute will you need to see the play button on your audio video in the browser?
controls HTML attribute you need to see the controls like play button, Pause button, Audio Seekbar and Volume Control button on your audio or video Element in the browser.
<audio src="song.mp3" controls></audio>
Make sure that your are using controls attribute inside the starting tag, anywhere after the tag name.
index.html
<audio src="https://programminghead.com/audio/audio.mp3" controls></audio>
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
Which HTML attribute will you need to see the play button on your audio video in the browser.
By default, audio and video controls are hidden inside our Web Browsers. So display the controls we need to use controls attribute inside our audio/video element's starting tag.
And this controls attribute will allow you to see the controls on your audio/video Element in the browser.
index.html
<audio src="https://programminghead.com/audio/audio.mp3" controls></audio>
Javascript play local audio file
To play local audio files using JavaScript, you will need to include audio files inside your HTML Document by using HTML Audio tags.
After that you need to add a ID to your Audio Tag (so you can select that HTML Element using JavaScript by the Element ID).
Add a HTML Button and by using onClick event inside HTML buttons we can call/Run JavaScript function.
Inside our JavaScript function select that Audio Element by using the ID and then use .play() to play the audio file.
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<button onClick="playMyAudio()">Play</button>
<script>
function playMyAudio(){
document.getElementById("myAudio").play();
}
</script>
</body>
</html>
Call a JavaScript function from HTML
To call a JavaScript function from HTML, we can use onClick event inside our HTML Elements.
So this onClick event will run the given JavaScript on getting clicked.
By using onClick event inside HTML Element we can call a JavaScript function from HTML.
index.html
<html>
<body>
<button onClick="runthis()">Run</button>
<script>
function runthis(){
alert("Using HTML");
}
</script>
</body>
</html>
Play audio in loop JavaScript
We can loop the audio in HTML by using JavaScript also without the JavaScript. So you can take a look at both JavaScript and HTML method and pick one that suits you.
Play audio in loop by using HTML [without JavaScript]
To loop an Audio in HTML we have loop attribute. By using loop attribute inside audio tag, we can tell our browser that we allow audio looping inside our HTML Document.
You can set loop="true" or just write loop inside the Audio tag, its going to work either way.
index.html
<audio src="mysong.mp3" controls loop></audio>
Play audio in loop [using JavaScript]
To loop audio by using JavaScript, we need to use Audio loop Property for that.
Where first we need to select our Audio Element by using document.getElementById("myAudio") and the using loop property we can set loop value true or false.
index.html
<html>
<body>
<audio src="audio.mp3" id="myAudio"></audio>
<script>
document.getElementById("myAudio").loop = true;
</script>
</body>
</html>
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.
Play mp3 in HTML
Nowadays, every web browser support .mp3 audio files. so you don't have to worry about browser compatibility.
You just need to make sure that your audio file and your HTML both are present in a same location or in a Same folder.
So you can easterly use it inside HTML Audio element and your browser can easily get the audio file inside your HTML Document.
If your audio file is present in a different location, then you need to give the full location. Because by default, web browser will look for that audio file in the same location where your HTML file is present.
To fix that either you can move your audio file and HTML file to same location/folder or you can use full audio path.
How to get Full audio Path?
To get the Full Path of your audio file you need open file Explorer > locate your Audio file > select > right click > properties > security.
There you will find your fill audio path that going to look like this ("C:/user/yourName/Downloads/song.mp3")
Copy that path and use it inside audio tag's SRC attribute (like <audio SRC="C:/user/yourName/Downloads/song.mp3" controls></audio>)
If you are still not able to load your audio file? then you can use file:/// before your audio file path and hopefully this time your audio file will work inside your HTML Document.
Still Not working - Facing Issues?
After trying everything, if its still not working then you can contact me using my email address programminghead7@gmail.com.
Just mail me your Problem in detail and we will fix the problem together.
On This Page
Need a Personal Tutor?
I am here to help you with your Programming journey. HTML, CSS, JavaScript, ReactJS, NextJS, C, C++, C#, SQL and more.